How to Test a Checkbox Is Checked Using Vue Test Utils
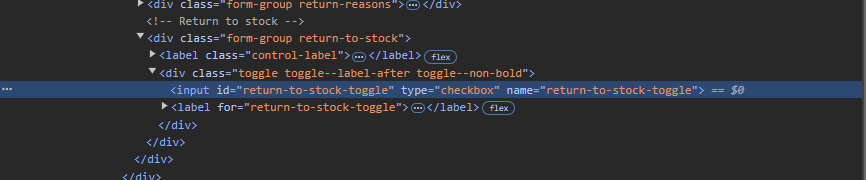
Say you want to test a Vue component that contains an HTML checkbox. When something happens the input should become checked.
The docs say that it sets a checked
property on the element. However in practise this didn't appear to work for me. I made a small demo component and test and then realised you need to check the [wrapper].element.checked
property, not just the [wrapper].checked
property.
Here's an example Vue component:
<template>
<div>
<input v-model="checked"
type="checkbox" />
</div>
</template>
<script>
export default {
data() {
return {
checked: false,
};
},
};
</script>
And a test:
import { mount } from '@vue/test-utils';
import ComponentWithCheckbox from './ComponentWithCheckbox';
describe('ComponentWithCheckbox', () => {
it('Should check the checkbox', async () => {
const wrapper = mount(ComponentWithCheckbox);
expect(wrapper.find('input[type="checkbox"]').element.checked)
.toBe(false);
await wrapper.setData({ checked: true });
expect(wrapper.find('input[type="checkbox"]').element.checked)
.toBe(true);
});
});
Notice that you call must add .element.checked
not just .checked